Purpose of this blog is to make programming easy , weak programmers may get help from my blog i tried my best to post some of my codes in form of easiest approach, I think this blog is perfect c++ guide in form of online blog. please consult my all codes and suggest all of it with your class mates. Share experiences & knowledge with your juniors. Make yourself habitual to write different programs for 2 hours a day daily.
Friday 10 October 2014
c++ print left triangle upper portion
void main()
{
int size=3;
cout<<"please enter the size of triangle to print";
cin>>size;
int margin_space;
int row=1;
while(row<=size)
{
margin_space = size - row;
int col = 0;
while(col<margin_space)
{
cout<<" ";
col = col+1;
}
int starics = 1;
while(starics<=row)
{
cout<<"X";
starics++;
}
cout<<endl;
row++;
}
{
int size=3;
cout<<"please enter the size of triangle to print";
cin>>size;
int margin_space;
int row=1;
while(row<=size)
{
margin_space = size - row;
int col = 0;
while(col<margin_space)
{
cout<<" ";
col = col+1;
}
int starics = 1;
while(starics<=row)
{
cout<<"X";
starics++;
}
cout<<endl;
row++;
}
}
output:-
Friday 25 July 2014
C++ Check Whether entered numbers are even or not
Purpose of the program is basically understanding that the entered various amount of numbers by user and test whether all of them are verifies that they are even number or not and how we check each number by using loop , what is the purpose to use loop why i use it ? efficient + reliable and deep understanding of concept make it possible to do efficient and complex less logic to easily understand program
Hope You will enjoy to do programming ....!
#include<iostream.h>
#include<conio.h>
int main()
{
int num1,num2,num3,num4;
for(int i=0;i<4;i++)
{
cout<<"Please Enter the number = "<<i<<" = ";
if(i==0){cin>>num1;}
else if(i==1){cin>>num2;}
else if(i==2){cin>>num3;}
else cin>>num4;
}
//cout<<"You have Entered the numbers are as under \n "<<num1<<"\n then = "<<num2<<"\n then = "<<num3<<"\n then ="<<num4<<endl;
cout<<"\n\t----Results-----";
cout<<"\n\t----Even or Odd-----";
cout<<"\n\n";
int i=0;
while(i<4)
{
if(i==0)
{
if(num1%2 == 0){ cout<<"Entered First number is even = "<<num1<<endl;}
else
cout<<"Entered First number is not even = "<<num1<<endl;
}
if(i==1)
{
if(num2%2 == 0){ cout<<"Entered second number is even = "<<num2<<endl;}
else
cout<<"Entered second number is not even = "<<num2<<endl;
}
if(i==2)
{
if(num3%2 == 0){ cout<<"Entered third number is even = "<<num3<<endl;}
else
cout<<"Entered third number is not even = "<<num3<<endl;
}
if(i==3)
{
if(num4%2 == 0){ cout<<"Entered Fourth number is even = "<<num4<<endl;}
else
cout<<"Entered Fourth number is not even = "<<num4<<endl;
}
i++;
}
getch();
return 0;
}
Sunday 1 June 2014
C++ Diamond Program using nested loop
#include"stdafx.h"
#include<iostream>
#include<conio.h>
using namespace std;
void main()
{
int space_margin;
int size=10;
int S_height;
char ch;
space_margin = 10;
do{
cout << "Enter the size of the diamond please = ? ";
cin >> size;
for (int i = 0; i <= size; i++)
{
S_height = space_margin + size - i;
for (int j = 0; j <= S_height; j++)
{
cout << ' ';
}
for (int j = 0; j <= i * 2; j++)
{
cout << "X";
}
cout << endl;
}
int sp;
for (int i = size; i >= 0; i--)
{
sp = S_height + size - i;
for (int j = sp; j >= 0; j--)
{
cout << ' ';
}
for (int j = i * 2; j >= 0; j--)
{
cout << "X";
}
cout << endl;
}
cout <<endl<<"Do you wish wants to contiue......Y/N"<<endl;
cin >> ch;
} while (ch == 'y' || ch == 'Y');
_getch();
Saturday 8 March 2014
C++ hollow diamond program
#include<iostream>
#include<conio.h>
using namespace std;
void main()
{
int Pheigt;
cout<<"Enter the height of paradigm:";
cin>>Pheigt;
int const margin_view = 20; //no need for constant it
int count;
int spaces_print;
int *ptr;
//**********************ROWS PRINT***************************************//
for(int i=0;i<=Pheigt;i++)
{
int spaces_to_print = margin_view +Pheigt - i; //*****************************//SETTING HOW MUCH SPACES TO PRINT
//cout<<spaces_to_print;
for(count = 0 ; count <= spaces_to_print; count++)
{
cout<<" ";
}
for(count = 0; count<=i*2; count++ ) //**********************PRINT STARS**************************//
{
if(count==0 || count==i*2)
{
cout<<"*";}
else{cout<<" ";}
}
ptr = &spaces_to_print;
//cout<<*ptr;
// cout<<spaces_to_print;
cout<<endl;
}
// cout<<*ptr;
int margins_view = *ptr; //current spaces store to new variable
//*************************Row Loop***********************************************//
for(int i=Pheigt;i>=0;i--)
{
spaces_print = margins_view + Pheigt - i;
// cout<<spaces_print;
for(count = spaces_print ; count >=0; count --)
{
cout<<" ";
}
for(count=i*2;count>=0;count--)
{
if(count==0 || count==i*2)
{
cout<<"*";}
else{cout<<" ";}
//cout<<"*";
}
//spaces
cout<<endl;
}
_getch();
}
Enter the height of paradigm:7
*
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
* *
*
C++ DIAMOND PROGRAM EASY APPROACH WITH FULL DESCRIPTION
QUESTIONS IN MIND
height is set because program does not know what to draw you have to pass the height of above and below pyramid that program can knew that height is etc height = 10; then it has to use logical height 10 times.
why nested loop ?
Always Remember C++ compiler only draw in form of rows and column a loop
nested means loop under a loop
ROW(PROGRAMMING){
_________COL(PROGRAMMING)
}
first loop is for rows (------------------------------)to print
2nd loop is for the column to print
(
|
|
|
)
now how to draw above and below pyramid??
Usually most of the time teachers and colleagues don't tell you what is logic to print it in easy way, i am experienced person so i will help you guys
1)ROWS
first of all you have to print pass the loop to compiler C++ which starts from 0 and ends to height you know
in which it now needs to know in a line single or line by line
you have to use \n or endl to print row by row a (loop prints row)
2) COLUMNS
As you see pyramid
*
***
*****
you notices their are spaces and margin in center and printing '*' in both sides
how
you have to define integer variable to set margin = for example 10
know
you have to store spaces for rows you see spaces are row by row reduced to help static print at left
int spaces = margin_you have given + height you have give - rows loop current value
your spaces for current loop row will be set
you have to print spaces for this row
until this row spaces value reached
_______________________________
you above see line it shows space of current row
Now you have to print *
use a loop goes to current row*2 value of loop and print *
initally 0 then one star will be print
now
you se
_______________________________*
loop continues
row incremented
colomn space decremented
stars in row incremented
___________________________*
__________________________***
_________________________*****
________________________*******
_______________________*********
______________________***********
How much height you want to draw diamond?
Want to draw in Center of screen to show beauty?
if you dont pass spaces loop what happens?
why Nested Loop is important in this program?
Many questions are produced in mind of new programmers I have solution
why nested loop ?
Always Remember C++ compiler only draw in form of rows and column a loop
nested means loop under a loop
ROW(PROGRAMMING){
_________COL(PROGRAMMING)
}
first loop is for rows (------------------------------)to print
2nd loop is for the column to print
(
|
|
|
)
now how to draw above and below pyramid??
Usually most of the time teachers and colleagues don't tell you what is logic to print it in easy way, i am experienced person so i will help you guys
1)ROWS
first of all you have to print pass the loop to compiler C++ which starts from 0 and ends to height you know
in which it now needs to know in a line single or line by line
you have to use \n or endl to print row by row a (loop prints row)
2) COLUMNS
As you see pyramid
*
***
*****
you notices their are spaces and margin in center and printing '*' in both sides
how
you have to define integer variable to set margin = for example 10
know
you have to store spaces for rows you see spaces are row by row reduced to help static print at left
int spaces = margin_you have given + height you have give - rows loop current value
your spaces for current loop row will be set
you have to print spaces for this row
until this row spaces value reached
_______________________________
you above see line it shows space of current row
Now you have to print *
use a loop goes to current row*2 value of loop and print *
initally 0 then one star will be print
now
you se
_______________________________*
loop continues
row incremented
colomn space decremented
stars in row incremented
___________________________*
__________________________***
_________________________*****
________________________*******
_______________________*********
______________________***********
till height is given
now reverse do process for print in reverse order how?
______________________***********
_______________________*********
________________________*******
_________________________*****
__________________________***
___________________________*
first of all samely you have to pass row for loop then space for loop in between row loop same as stars loop
how you pass the space margin to other row for loop you have to use pointer for which you can get the margin space total gone and last time used for upper paramid now you can by using pointer save in it last value of margin space of pointer and pass to lower margin space pointer so which from you for loop row starts and ends to 0 to print in reverse order
in upper row for loop pass this code
ptr = &spaces_to_print;
it can saves last pointer then
after end of loop declare another space margin variable and pass this pointer to it and start the loop from it and till ends to 0
int margins_view = *ptr;
for(int i=Pheigt;i>=0;i--)
{
// your code
}
same above procedure but in reverse
spaces_print = margins_view + Pheigt - i;
and you have got upper and lower paramid form diamond i hope you will understand my code
now reverse do process for print in reverse order how?
______________________***********
_______________________*********
________________________*******
_________________________*****
__________________________***
___________________________*
first of all samely you have to pass row for loop then space for loop in between row loop same as stars loop
how you pass the space margin to other row for loop you have to use pointer for which you can get the margin space total gone and last time used for upper paramid now you can by using pointer save in it last value of margin space of pointer and pass to lower margin space pointer so which from you for loop row starts and ends to 0 to print in reverse order
in upper row for loop pass this code
ptr = &spaces_to_print;
it can saves last pointer then
after end of loop declare another space margin variable and pass this pointer to it and start the loop from it and till ends to 0
int margins_view = *ptr;
for(int i=Pheigt;i>=0;i--)
{
// your code
}
same above procedure but in reverse
spaces_print = margins_view + Pheigt - i;
and you have got upper and lower paramid form diamond i hope you will understand my code
- #include<iostream>
- #include<conio.h>
- using namespace std;
- void main()
- {
- int Pheigt;
- cout<<"Enter the height of paradigm:";
- cin>>Pheigt;
- int const margin_view = 20; //no need for constant it
- int count;
- int spaces_print;
- int *ptr;
- //**********************ROWS PRINT***************************************//
- for(int i=0;i<=Pheigt;i++)
- {
- int spaces_to_print = margin_view +Pheigt - i; //*****************************//SETTING HOW MUCH SPACES TO PRINT
- //cout<<spaces_to_print;
- for(count = 0 ; count <= spaces_to_print; count++)
- {
- cout<<" ";
- }
- for(count = 0; count<=i*2; count++ ) //**********************PRINT STARS**************************//
- {
- cout<<"*";
- }
- ptr = &spaces_to_print;
- //cout<<*ptr;
- // cout<<spaces_to_print;
- cout<<endl;
- }
- // cout<<*ptr;
- int margins_view = *ptr;
- for(int i=Pheigt;i>=0;i--)
- {
- spaces_print = margins_view + Pheigt - i;
- // cout<<spaces_print;
- for(count = spaces_print ; count >=0; count --)
- {
- cout<<" ";
- }
- for(count=i*2;count>=0;count--)
- {
- cout<<"*";
- }
- //spaces
- cout<<endl;
- }
- _getch();
- }
Thursday 6 March 2014
C++ FULL PARAMID PROGRAM EASIEST APPROACH
QUESTIONS IN MIND
height is set because program does not know what to draw you have to pass the height of pyramid that program can knew that height is etc height = 10; then it has to use logical height 10 times.
why nested loop ?
Always Remember C++ compiler only draw in form of rows and column a loop
nested means loop under a loop
ROW(PROGRAMMING){
_________COL(PROGRAMMING)
}
first loop is for rows (------------------------------)to print
2nd loop is for the column to print
(
|
|
|
)
now how to draw pyramid??
Usually most of the time teachers and colleagues don't tell you what is logic to print it in easy way, i am experienced person so i will help you guys
1)ROWS
first of all you have to print pass the loop to compiler C++ which starts from 0 and ends to height you know
in which it now needs to know in a line single or line by line
you have to use \n or endl to print row by row a (loop prints row)
2) COLUMNS
As you see pyramid
*
***
*****
you notices their are spaces and margin in center and printing '*' in both sides
how
you have to define integer variable to set margin = for example 10
know
you have to store spaces for rows you see spaces are row by row reduced to help static print at left
int spaces = margin_you have given + height you have give - rows loop current value
your spaces for current loop row will be set
you have to print spaces for this row
until this row spaces value reached
_______________________________
you above see line it shows space of current row
Now you have to print *
use a loop goes to current row*2 value of loop and print *
initally 0 then one star will be print
now
you se
_______________________________*
loop continues
row incremented
colomn space decremented
stars in row incremented
___________________________*
__________________________***
_________________________*****
________________________*******
_______________________*********
______________________***********
How much height you want to draw paramid?
Want to draw in Center of screen to show beauty?
if you dont pass spaces loop what happens?
why Nested Loop is important in this program?
Many questions are produced in mind of new programmers I have solution
why nested loop ?
Always Remember C++ compiler only draw in form of rows and column a loop
nested means loop under a loop
ROW(PROGRAMMING){
_________COL(PROGRAMMING)
}
first loop is for rows (------------------------------)to print
2nd loop is for the column to print
(
|
|
|
)
now how to draw pyramid??
Usually most of the time teachers and colleagues don't tell you what is logic to print it in easy way, i am experienced person so i will help you guys
1)ROWS
first of all you have to print pass the loop to compiler C++ which starts from 0 and ends to height you know
in which it now needs to know in a line single or line by line
you have to use \n or endl to print row by row a (loop prints row)
2) COLUMNS
As you see pyramid
*
***
*****
you notices their are spaces and margin in center and printing '*' in both sides
how
you have to define integer variable to set margin = for example 10
know
you have to store spaces for rows you see spaces are row by row reduced to help static print at left
int spaces = margin_you have given + height you have give - rows loop current value
your spaces for current loop row will be set
you have to print spaces for this row
until this row spaces value reached
_______________________________
you above see line it shows space of current row
Now you have to print *
use a loop goes to current row*2 value of loop and print *
initally 0 then one star will be print
now
you se
_______________________________*
loop continues
row incremented
colomn space decremented
stars in row incremented
___________________________*
__________________________***
_________________________*****
________________________*******
_______________________*********
______________________***********
till height is given
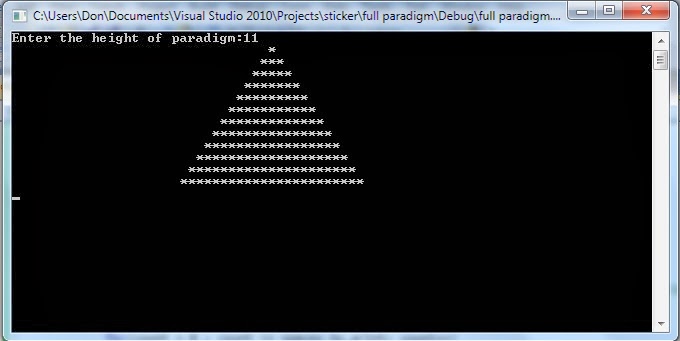
//CODE
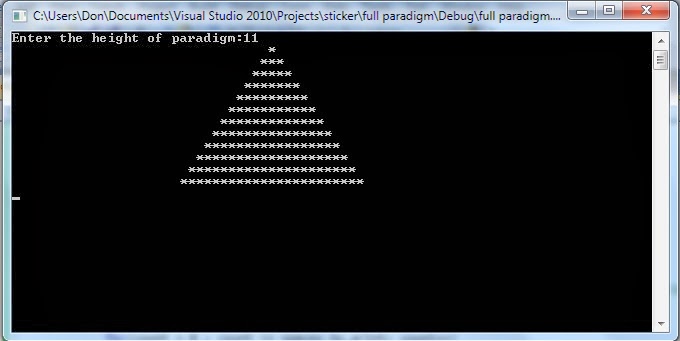
//CODE
#include<iostream>
#include<conio.h>
using namespace std;
void main()
{
int Pheigt;
cout<<"Enter the height of paradigm:";
cin>>Pheigt;
int margin_view = 20; //no need for constant it
int count;
//**********************ROWS PRINT***************************************//
for(int i=0;i<=Pheigt;i++)
{
int spaces_to_print = margin_view +Pheigt - i; //*****************************//SETTING HOW MUCH SPACES TO PRINT
for(count = 0 ; count <= spaces_to_print; count++)
{
cout<<" ";
}
for(count = 0; count<=i*2; count++ ) //**********************PRINT STARS**************************//
{
cout<<"*";
}
// cout<<spaces_to_print;
cout<<endl;
}
_getch();
}
Thursday 27 February 2014
C++ MATRIX ADD SUBTRACT MULTIPLY DIVIDE OPERATION AND FIND MAXIMUM MINIMUM VALUE IN MATRIX
//CODE
// we make 3 X 4 matrix
/***********************************************************************************/
#include<iostream>
#include<stdlib.h>
#include<cmath>
using namespace std;
void main()
{
int Matris[3][4];
int j=0;
int max_col,max_row,min_col,min_row;
/**************insert in matrics**************************/
int i=0;
while(i<3)//rows<#
{
while(j<4)
{
cout<<"enter the value of the row and colomn: ["<<i<<"]["<<j<<"]"<<endl;
cin>>Matris[i][j];
j++;
}
j=0;
i++;
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
/************Showing values in matrices********************/
cout<<endl<<"----------Values Are in Matrices as under----------"<<endl<<endl;
for(int m=0;m<3;m++)
{
for(int f=0;f<4;f++)
{
if(f==0)
{
// cout<<"Value Stored in row and colomn of Matric ["<<m<<"]["<<f<<"]"<<" IS : "<<Matris[m][f]<<endl;
cout<<Matris[m][f];
}
if(f!=0 && f!=3)
{
cout<<"\t"<<Matris[m][f];
}
if(f==3)
{
cout<<"\t"<<Matris[m][f]<<endl;
}
}
}
/************Decleration of new datatype to find maximum and minimum********************/
int max,min;
max = min = Matris[0][0];
cout<<endl<<"\n----------Finding maximum and minimum values----------"<<endl<<endl;
//less check
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
for(int p=0;p<3;p++){
for(int l=0;l<4;l++){
{
if(min>Matris[p][l])
{
min = Matris[p][l];
min_col = p;
min_row = l;
}
if(max<Matris[p][l])
{
max = Matris[p][l];
max_col = p;
max_row = l;
}}
}
}
cout<<"Maximum value found in matrices is : "<<Matris[max_col][max_row]<<endl;
cout<<"Minimum value found in matrices is : "<<Matris[min_col][min_row]<<endl;
/************************SECOND MATRICS*************************************************/
int Matris2[3][4];
i=0,j=0;
while(i<3)//rows<#
{
while(j<4)
{
cout<<"enter the value of the row and colomn: ["<<i<<"]["<<j<<"]"<<endl;
cin>>Matris2[i][j];
j++;
}
j=0;
i++;
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
cout<<endl<<"----------Values Are in Matrices # 2 as under----------"<<endl<<endl;
for(int m=0;m<3;m++)
{
for(int f=0;f<4;f++)
{
if(f==0)
{
cout<<Matris2[m][f];
}
if(f!=0 && f!=3)
{
cout<<"\t"<<Matris2[m][f];
}
if(f==3)
{
cout<<"\t"<<Matris2[m][f]<<endl;
}
}
}
max = min = Matris2[0][0];
for(int p=0;p<3;p++){
for(int l=0;l<4;l++){
{
if(min>Matris2[p][l])
{
min = Matris2[p][l];
min_col = p;
min_row = l;
}
if(max<Matris2[p][l])
{
max = Matris[p][l];
max_col = p;
max_row = l;
}}
}
}
cout<<"Maximum value found in matrices is : "<<Matris2[max_col][max_row]<<endl;
cout<<"Minimum value found in matrices is : "<<Matris2[min_col][min_row]<<endl;
/************************SUM OF MATRICS****************************************************/
int sumMatrics[3][4];
for(int z=0;z<3;z++)
{
for(int x=0;x<4;x++)
{
sumMatrics[z][x] = Matris[z][x] + Matris2[z][x];
}
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
cout<<endl<<"----------Values Are in SUM MATRICS as under----------"<<endl<<endl;
for(int m=0;m<3;m++)
{
for(int f=0;f<4;f++)
{
if(f==0)
{
cout<<sumMatrics[m][f];
}
if(f!=0 && f!=3)
{
cout<<"\t"<<sumMatrics[m][f];
}
if(f==3)
{
cout<<"\t"<<sumMatrics[m][f]<<endl;
}
}
}
max = min = sumMatrics[0][0];
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
for(int p=0;p<3;p++)
{
for(int l=0;l<4;l++)
{
{
if(min>sumMatrics[p][l])
{
min = sumMatrics[p][l];
min_col = p;
min_row = l;
}
if(max<sumMatrics[p][l])
{
max = sumMatrics[p][l];
max_col = p;
max_row = l;
}
}
}
}
cout<<"Maximum value found in matrices is : "<<sumMatrics[max_col][max_row]<<endl;
cout<<"Minimum value found in matrices is : "<<sumMatrics[min_col][min_row]<<endl;
/************************SUBTRACTION OF MATRICS****************************************************/
int subMatrics[3][4];
for(int z=0;z<3;z++)
{
for(int x=0;x<4;x++)
{
if( Matris2[z][x]>Matris[z][x]){
subMatrics[z][x] = Matris2[z][x] - Matris[z][x];}
else
{subMatrics[z][x] = Matris[z][x]-Matris2[z][x] ;}
}
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
cout<<endl<<"----------Values Are in SUM MATRICS as under----------"<<endl<<endl;
for(int m=0;m<3;m++)
{
for(int f=0;f<4;f++)
{
if(f==0)
{
cout<<subMatrics[m][f];
}
if(f!=0 && f!=3)
{
cout<<"\t"<<subMatrics[m][f];
}
if(f==3)
{
cout<<"\t"<<subMatrics[m][f]<<endl;
}
}
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
max = min = subMatrics[0][0];
for(int p=0;p<3;p++){
for(int l=0;l<4;l++){
{
if(min>subMatrics[p][l])
{
min = subMatrics[p][l];
min_col = p;
min_row = l;
}
if(max<subMatrics[p][l])
{
max = subMatrics[p][l];
max_col = p;
max_row = l;
}}
}
}
cout<<"Maximum value found in matrices is : "<<subMatrics[max_col][max_row]<<endl;
cout<<"Minimum value found in matrices is : "<<subMatrics[min_col][min_row]<<endl;
/************************Multiplication OF MATRICS****************************************************/
int multMatrics[3][4];
for(int z=0;z<3;z++)
{
for(int x=0;x<4;x++)
{
multMatrics[z][x] = Matris2[z][x] * Matris[z][x];
}
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
cout<<endl<<"----------Values Are in MULTIPLY MATRICS as under----------"<<endl<<endl;
for(int m=0;m<3;m++)
{
for(int f=0;f<4;f++)
{
if(f==0)
{
cout<<multMatrics[m][f];
}
if(f!=0 && f!=3)
{
cout<<"\t"<<multMatrics[m][f];
}
if(f==3)
{
cout<<"\t"<<multMatrics[m][f]<<endl;
}
}
}
max = min = multMatrics[0][0];
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
for(int p=0;p<3;p++){
for(int l=0;l<4;l++){
{
if(min>multMatrics[p][l])
{
min = multMatrics[p][l];
min_col = p;
min_row = l;
}
if(max<multMatrics[p][l])
{
max = multMatrics[p][l];
max_col = p;
max_row = l;
}}
}
}
cout<<"Maximum value found in matrices is : "<<multMatrics[max_col][max_row]<<endl;
cout<<"Minimum value found in matrices is : "<<multMatrics[min_col][min_row]<<endl;
/************************DIVISION OF MATRICS****************************************************/
int divMatrics[3][4];
for(int z=0;z<3;z++)
{
for(int x=0;x<4;x++)
{
divMatrics[z][x] = Matris2[z][x] / Matris[z][x];
}
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
cout<<endl<<"----------Values Are in DIVISION MATRICS as under----------"<<endl<<endl;
for(int m=0;m<3;m++)
{
for(int f=0;f<4;f++)
{
if(f==0)
{
cout<<divMatrics[m][f];
}
if(f!=0 && f!=3)
{
cout<<"\t"<<divMatrics[m][f];
}
if(f==3)
{
cout<<"\t"<<divMatrics[m][f]<<endl;
}
}
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
max = min = divMatrics[0][0];
for(int p=0;p<3;p++){
for(int l=0;l<4;l++){
{
if(min>divMatrics[p][l])
{
min = divMatrics[p][l];
min_col = p;
min_row = l;
}
if(max<divMatrics[p][l])
{
max = divMatrics[p][l];
max_col = p;
max_row = l;
}}
}
}
cout<<"Maximum value found in matrices is : "<<divMatrics[max_col][max_row]<<endl;
cout<<"Minimum value found in matrices is : "<<divMatrics[min_col][min_row]<<endl;
cout<<"\n\n\t\t\t PRogRam PRO C++ (UMAR JAVED)"<<endl;
cout<<"\n\n\n\t\t\t***************************"<<endl;
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
int h;
cin>>h;
}
/**************************output**********************************/
//enter the value of the row and colomn: [0][0]
//5
//enter the value of the row and colomn: [0][1]
//1
//enter the value of the row and colomn: [0][2]
//2
//enter the value of the row and colomn: [0][3]
//6
//enter the value of the row and colomn: [1][0]
//11
//enter the value of the row and colomn: [1][1]
//7
//enter the value of the row and colomn: [1][2]
//2
//enter the value of the row and colomn: [1][3]
//6
//enter the value of the row and colomn: [2][0]
//4
//enter the value of the row and colomn: [2][1]
//3
//enter the value of the row and colomn: [2][2]
//8
//enter the value of the row and colomn: [2][3]
//9
//
//----------Values Are in Matrices as under----------
//
//5 1 2 6
//11 7 2 6
//4 3 8 9
//
//
//----------Finding maximum and minimum values----------
//
//Maximum value found in matrices is : 11
//Minimum value found in matrices is : 1
//enter the value of the row and colomn: [0][0]
//11
//enter the value of the row and colomn: [0][1]
//52
//enter the value of the row and colomn: [0][2]
//65
//enter the value of the row and colomn: [0][3]
//75
//enter the value of the row and colomn: [1][0]
//32
//enter the value of the row and colomn: [1][1]
//21
//enter the value of the row and colomn: [1][2]
//25
//enter the value of the row and colomn: [1][3]
//85
//enter the value of the row and colomn: [2][0]
//75
//enter the value of the row and colomn: [2][1]
//65
//enter the value of the row and colomn: [2][2]
//12
//enter the value of the row and colomn: [2][3]
//32
//
//----------Values Are in Matrices # 2 as under----------
//
//11 52 65 75
//32 21 25 85
//75 65 12 32
//Maximum value found in matrices is : 32
//Minimum value found in matrices is : 52
//
//----------Values Are in SUM MATRICS as under----------
//
//16 53 67 81
//43 28 27 91
//79 68 20 41
//Maximum value found in matrices is : 91
//Minimum value found in matrices is : 53
//
//----------Values Are in SUM MATRICS as under----------
//
//6 51 63 69
//21 14 23 79
//71 62 4 23
//Maximum value found in matrices is : 79
//Minimum value found in matrices is : 4
//
//----------Values Are in MULTIPLY MATRICS as under----------
//
//55 52 130 450
//352 147 50 510
//300 195 96 288
//Maximum value found in matrices is : 510
//Minimum value found in matrices is : 50
//
//----------Values Are in DIVISION MATRICS as under----------
//
//2 52 32 12
//2 3 12 14
//18 21 1 3
//Maximum value found in matrices is : 52
//Minimum value found in matrices is : 1
// we make 3 X 4 matrix
/***********************************************************************************/
#include<iostream>
#include<stdlib.h>
#include<cmath>
using namespace std;
void main()
{
int Matris[3][4];
int j=0;
int max_col,max_row,min_col,min_row;
/**************insert in matrics**************************/
int i=0;
while(i<3)//rows<#
{
while(j<4)
{
cout<<"enter the value of the row and colomn: ["<<i<<"]["<<j<<"]"<<endl;
cin>>Matris[i][j];
j++;
}
j=0;
i++;
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
/************Showing values in matrices********************/
cout<<endl<<"----------Values Are in Matrices as under----------"<<endl<<endl;
for(int m=0;m<3;m++)
{
for(int f=0;f<4;f++)
{
if(f==0)
{
// cout<<"Value Stored in row and colomn of Matric ["<<m<<"]["<<f<<"]"<<" IS : "<<Matris[m][f]<<endl;
cout<<Matris[m][f];
}
if(f!=0 && f!=3)
{
cout<<"\t"<<Matris[m][f];
}
if(f==3)
{
cout<<"\t"<<Matris[m][f]<<endl;
}
}
}
/************Decleration of new datatype to find maximum and minimum********************/
int max,min;
max = min = Matris[0][0];
cout<<endl<<"\n----------Finding maximum and minimum values----------"<<endl<<endl;
//less check
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
for(int p=0;p<3;p++){
for(int l=0;l<4;l++){
{
if(min>Matris[p][l])
{
min = Matris[p][l];
min_col = p;
min_row = l;
}
if(max<Matris[p][l])
{
max = Matris[p][l];
max_col = p;
max_row = l;
}}
}
}
cout<<"Maximum value found in matrices is : "<<Matris[max_col][max_row]<<endl;
cout<<"Minimum value found in matrices is : "<<Matris[min_col][min_row]<<endl;
/************************SECOND MATRICS*************************************************/
int Matris2[3][4];
i=0,j=0;
while(i<3)//rows<#
{
while(j<4)
{
cout<<"enter the value of the row and colomn: ["<<i<<"]["<<j<<"]"<<endl;
cin>>Matris2[i][j];
j++;
}
j=0;
i++;
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
cout<<endl<<"----------Values Are in Matrices # 2 as under----------"<<endl<<endl;
for(int m=0;m<3;m++)
{
for(int f=0;f<4;f++)
{
if(f==0)
{
cout<<Matris2[m][f];
}
if(f!=0 && f!=3)
{
cout<<"\t"<<Matris2[m][f];
}
if(f==3)
{
cout<<"\t"<<Matris2[m][f]<<endl;
}
}
}
max = min = Matris2[0][0];
for(int p=0;p<3;p++){
for(int l=0;l<4;l++){
{
if(min>Matris2[p][l])
{
min = Matris2[p][l];
min_col = p;
min_row = l;
}
if(max<Matris2[p][l])
{
max = Matris[p][l];
max_col = p;
max_row = l;
}}
}
}
cout<<"Maximum value found in matrices is : "<<Matris2[max_col][max_row]<<endl;
cout<<"Minimum value found in matrices is : "<<Matris2[min_col][min_row]<<endl;
/************************SUM OF MATRICS****************************************************/
int sumMatrics[3][4];
for(int z=0;z<3;z++)
{
for(int x=0;x<4;x++)
{
sumMatrics[z][x] = Matris[z][x] + Matris2[z][x];
}
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
cout<<endl<<"----------Values Are in SUM MATRICS as under----------"<<endl<<endl;
for(int m=0;m<3;m++)
{
for(int f=0;f<4;f++)
{
if(f==0)
{
cout<<sumMatrics[m][f];
}
if(f!=0 && f!=3)
{
cout<<"\t"<<sumMatrics[m][f];
}
if(f==3)
{
cout<<"\t"<<sumMatrics[m][f]<<endl;
}
}
}
max = min = sumMatrics[0][0];
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
for(int p=0;p<3;p++)
{
for(int l=0;l<4;l++)
{
{
if(min>sumMatrics[p][l])
{
min = sumMatrics[p][l];
min_col = p;
min_row = l;
}
if(max<sumMatrics[p][l])
{
max = sumMatrics[p][l];
max_col = p;
max_row = l;
}
}
}
}
cout<<"Maximum value found in matrices is : "<<sumMatrics[max_col][max_row]<<endl;
cout<<"Minimum value found in matrices is : "<<sumMatrics[min_col][min_row]<<endl;
/************************SUBTRACTION OF MATRICS****************************************************/
int subMatrics[3][4];
for(int z=0;z<3;z++)
{
for(int x=0;x<4;x++)
{
if( Matris2[z][x]>Matris[z][x]){
subMatrics[z][x] = Matris2[z][x] - Matris[z][x];}
else
{subMatrics[z][x] = Matris[z][x]-Matris2[z][x] ;}
}
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
cout<<endl<<"----------Values Are in SUM MATRICS as under----------"<<endl<<endl;
for(int m=0;m<3;m++)
{
for(int f=0;f<4;f++)
{
if(f==0)
{
cout<<subMatrics[m][f];
}
if(f!=0 && f!=3)
{
cout<<"\t"<<subMatrics[m][f];
}
if(f==3)
{
cout<<"\t"<<subMatrics[m][f]<<endl;
}
}
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
max = min = subMatrics[0][0];
for(int p=0;p<3;p++){
for(int l=0;l<4;l++){
{
if(min>subMatrics[p][l])
{
min = subMatrics[p][l];
min_col = p;
min_row = l;
}
if(max<subMatrics[p][l])
{
max = subMatrics[p][l];
max_col = p;
max_row = l;
}}
}
}
cout<<"Maximum value found in matrices is : "<<subMatrics[max_col][max_row]<<endl;
cout<<"Minimum value found in matrices is : "<<subMatrics[min_col][min_row]<<endl;
/************************Multiplication OF MATRICS****************************************************/
int multMatrics[3][4];
for(int z=0;z<3;z++)
{
for(int x=0;x<4;x++)
{
multMatrics[z][x] = Matris2[z][x] * Matris[z][x];
}
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
cout<<endl<<"----------Values Are in MULTIPLY MATRICS as under----------"<<endl<<endl;
for(int m=0;m<3;m++)
{
for(int f=0;f<4;f++)
{
if(f==0)
{
cout<<multMatrics[m][f];
}
if(f!=0 && f!=3)
{
cout<<"\t"<<multMatrics[m][f];
}
if(f==3)
{
cout<<"\t"<<multMatrics[m][f]<<endl;
}
}
}
max = min = multMatrics[0][0];
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
for(int p=0;p<3;p++){
for(int l=0;l<4;l++){
{
if(min>multMatrics[p][l])
{
min = multMatrics[p][l];
min_col = p;
min_row = l;
}
if(max<multMatrics[p][l])
{
max = multMatrics[p][l];
max_col = p;
max_row = l;
}}
}
}
cout<<"Maximum value found in matrices is : "<<multMatrics[max_col][max_row]<<endl;
cout<<"Minimum value found in matrices is : "<<multMatrics[min_col][min_row]<<endl;
/************************DIVISION OF MATRICS****************************************************/
int divMatrics[3][4];
for(int z=0;z<3;z++)
{
for(int x=0;x<4;x++)
{
divMatrics[z][x] = Matris2[z][x] / Matris[z][x];
}
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
cout<<endl<<"----------Values Are in DIVISION MATRICS as under----------"<<endl<<endl;
for(int m=0;m<3;m++)
{
for(int f=0;f<4;f++)
{
if(f==0)
{
cout<<divMatrics[m][f];
}
if(f!=0 && f!=3)
{
cout<<"\t"<<divMatrics[m][f];
}
if(f==3)
{
cout<<"\t"<<divMatrics[m][f]<<endl;
}
}
}
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
max = min = divMatrics[0][0];
for(int p=0;p<3;p++){
for(int l=0;l<4;l++){
{
if(min>divMatrics[p][l])
{
min = divMatrics[p][l];
min_col = p;
min_row = l;
}
if(max<divMatrics[p][l])
{
max = divMatrics[p][l];
max_col = p;
max_row = l;
}}
}
}
cout<<"Maximum value found in matrices is : "<<divMatrics[max_col][max_row]<<endl;
cout<<"Minimum value found in matrices is : "<<divMatrics[min_col][min_row]<<endl;
cout<<"\n\n\t\t\t PRogRam PRO C++ (UMAR JAVED)"<<endl;
cout<<"\n\n\n\t\t\t***************************"<<endl;
/*************************COPY RIGHTS TO PROGRAMMINGISBEST/{UMARJAVED01@GMAIL.COM}*********************************************************/
int h;
cin>>h;
}
/**************************output**********************************/
//enter the value of the row and colomn: [0][0]
//5
//enter the value of the row and colomn: [0][1]
//1
//enter the value of the row and colomn: [0][2]
//2
//enter the value of the row and colomn: [0][3]
//6
//enter the value of the row and colomn: [1][0]
//11
//enter the value of the row and colomn: [1][1]
//7
//enter the value of the row and colomn: [1][2]
//2
//enter the value of the row and colomn: [1][3]
//6
//enter the value of the row and colomn: [2][0]
//4
//enter the value of the row and colomn: [2][1]
//3
//enter the value of the row and colomn: [2][2]
//8
//enter the value of the row and colomn: [2][3]
//9
//
//----------Values Are in Matrices as under----------
//
//5 1 2 6
//11 7 2 6
//4 3 8 9
//
//
//----------Finding maximum and minimum values----------
//
//Maximum value found in matrices is : 11
//Minimum value found in matrices is : 1
//enter the value of the row and colomn: [0][0]
//11
//enter the value of the row and colomn: [0][1]
//52
//enter the value of the row and colomn: [0][2]
//65
//enter the value of the row and colomn: [0][3]
//75
//enter the value of the row and colomn: [1][0]
//32
//enter the value of the row and colomn: [1][1]
//21
//enter the value of the row and colomn: [1][2]
//25
//enter the value of the row and colomn: [1][3]
//85
//enter the value of the row and colomn: [2][0]
//75
//enter the value of the row and colomn: [2][1]
//65
//enter the value of the row and colomn: [2][2]
//12
//enter the value of the row and colomn: [2][3]
//32
//
//----------Values Are in Matrices # 2 as under----------
//
//11 52 65 75
//32 21 25 85
//75 65 12 32
//Maximum value found in matrices is : 32
//Minimum value found in matrices is : 52
//
//----------Values Are in SUM MATRICS as under----------
//
//16 53 67 81
//43 28 27 91
//79 68 20 41
//Maximum value found in matrices is : 91
//Minimum value found in matrices is : 53
//
//----------Values Are in SUM MATRICS as under----------
//
//6 51 63 69
//21 14 23 79
//71 62 4 23
//Maximum value found in matrices is : 79
//Minimum value found in matrices is : 4
//
//----------Values Are in MULTIPLY MATRICS as under----------
//
//55 52 130 450
//352 147 50 510
//300 195 96 288
//Maximum value found in matrices is : 510
//Minimum value found in matrices is : 50
//
//----------Values Are in DIVISION MATRICS as under----------
//
//2 52 32 12
//2 3 12 14
//18 21 1 3
//Maximum value found in matrices is : 52
//Minimum value found in matrices is : 1
Subscribe to:
Posts (Atom)